点击上方“菜学Python”,选择“星标”公众号
超级无敌干货,第一时间送达!!!
水果忍者的玩法很简单,尽可能的切开抛出的水果就行。
今天小五就用python简单的模拟一下这个游戏。在这个简单的项目中,我们用鼠标选择水果来切割,同时炸弹也会隐藏在水果中,如果切开了三次炸弹,玩家就会失败。
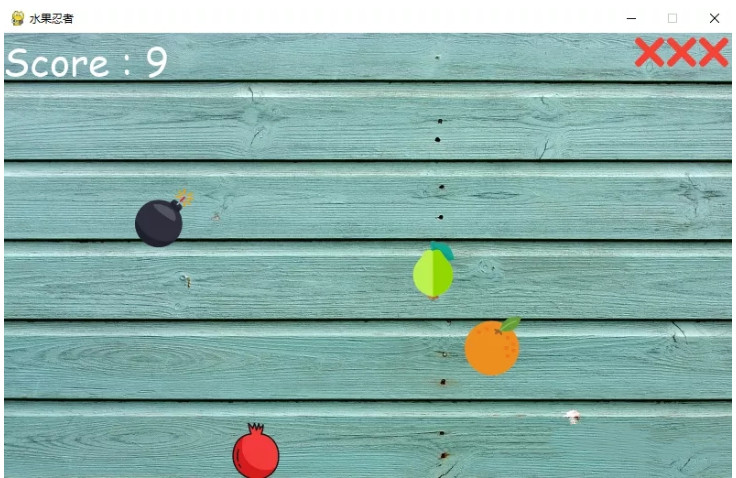
一、需要导入的包
import pygame, sys
import os
import random
二、窗口界面设置
# 游戏窗口
WIDTH = 800
HEIGHT = 500
FPS = 15 # gameDisplay的帧率,1/12秒刷新一次
pygame.init()
pygame.display.set_caption('水果忍者') # 标题
gameDisplay = pygame.display.set_mode((WIDTH, HEIGHT)) # 固定窗口大小
clock = pygame.time.Clock()
# 用到的颜色
WHITE = (255,255,255)
BLACK = (0,0,0)
RED = (255,0,0)
GREEN = (0,255,0)
BLUE = (0,0,255)
background = pygame.image.load('背景.jpg') # 背景
font = pygame.font.Font(os.path.join(os.getcwd(), 'comic.ttf'), 42) # 字体
score_text = font.render('Score : ' + str(score), True, (255, 255, 255)) # 得分字体样式
三、随机生成水果位置
def generate_random_fruits(fruit):
fruit_path = "images/" + fruit + ".png"
data[fruit] = {
'img': pygame.image.load(fruit_path),
'x' : random.randint(100,500),
'y' : 800,
'speed_x': random.randint(-10,10),
'speed_y': random.randint(-80, -60),
'throw': False,
't': 0,
'hit': False,
}
if random.random() >= 0.75:
data[fruit]['throw'] = True
else:
data[fruit]['throw'] = False
data = {}
for fruit in fruits:
generate_random_fruits(fruit)
四、绘制字体
font_name = pygame.font.match_font('comic.ttf')
def draw_text(display, text, size, x, y):
font = pygame.font.Font(font_name, size)
text_surface = font.render(text, True, WHITE)
text_rect = text_surface.get_rect()
text_rect.midtop = (x, y)
gameDisplay.blit(text_surface, text_rect)
五、玩家生命的提示
# 绘制玩家的生命
def draw_lives(display, x, y, lives, image) :
for i in range(lives) :
img = pygame.image.load(image)
img_rect = img.get_rect()
img_rect.x = int(x + 35 * i)
img_rect.y = y
display.blit(img, img_rect)
def hide_cross_lives(x, y):
gameDisplay.blit(pygame.image.load("images/red_lives.png"), (x, y))
img_rect获取十字图标的(x,y)坐标(位于最右上方)
img_rect .x 设置下一个十字图标距离前一个图标35像素。
img_rect.y负责确定十字图标从屏幕顶部开始的位置。
六、游戏开始与结束的画面
def show_gameover_screen():
gameDisplay.blit(background, (0,0))
draw_text(gameDisplay, "FRUIT NINJA!", 90, WIDTH / 2, HEIGHT / 4)
if not game_over :
draw_text(gameDisplay,"Score : " + str(score), 50, WIDTH / 2, HEIGHT /2)
draw_text(gameDisplay, "Press a key to begin!", 64, WIDTH / 2, HEIGHT * 3 / 4)
pygame.display.flip()
waiting = True
while waiting:
clock.tick(FPS)
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
if event.type == pygame.KEYUP:
waiting = False
show_gameover_screen()函数显示初始游戏画面和游戏结束画面。
pygame.display.flip()将只更新屏幕的一部分,但如果没有参数传递,则会更新整个屏幕。
pygame.event.get()将返回存储在pygame事件队列中的所有事件。
如果事件类型等于quit,那么pygame将退出。
event.KEYUP事件,当按键被按下和释放时发生的事件。
七、游戏主循环
first_round = True
game_over = True
game_running = True
while game_running :
if game_over :
if first_round :
show_gameover_screen()
first_round = False
game_over = False
player_lives = 3
draw_lives(gameDisplay, 690, 5, player_lives, 'images/red_lives.png')
score = 0
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_running = False
gameDisplay.blit(background, (0, 0))
gameDisplay.blit(score_text, (0, 0))
draw_lives(gameDisplay, 690, 5, player_lives, 'images/red_lives.png')
for key, value in data.items():
if value['throw']:
value['x'] += value['speed_x']
value['y'] += value['speed_y']
value['speed_y'] += (1 * value['t'])
value['t'] += 1
if value['y'] <= 800:
gameDisplay.blit(value['img'], (value['x'], value['y']))
else:
generate_random_fruits(key)
current_position = pygame.mouse.get_pos()
if not value['hit'] and current_position[0] > value['x'] and current_position[0] < value['x']+60 \
and current_position[1] > value['y'] and current_position[1] < value['y']+60:
if key == 'bomb':
player_lives -= 1
if player_lives == 0:
hide_cross_lives(690, 15)
elif player_lives == 1 :
hide_cross_lives(725, 15)
elif player_lives == 2 :
hide_cross_lives(760, 15)
if player_lives < 0 :
show_gameover_screen()
game_over = True
half_fruit_path = "images/explosion.png"
else:
half_fruit_path = "images/" + "half_" + key + ".png"
value['img'] = pygame.image.load(half_fruit_path)
value['speed_x'] += 10
if key != 'bomb' :
score += 1
score_text = font.render('Score : ' + str(score), True, (255, 255, 255))
value['hit'] = True
else:
generate_random_fruits(key)
pygame.display.update()
clock.tick(FPS)
pygame.quit()
这是游戏的主循环
如果超过3个炸弹被切掉,game_over终止游戏,同时循环。
game_running 用于管理游戏循环。
如果事件类型是退出,那么游戏窗口将被关闭。
在这个游戏循环中,我们动态显示屏幕内的水果。
如果一个水果没有被切开,那么它将不会发生任何事情。如果水果被切开,那么一个半切开的水果图像应该出现在该水果的地方
如果用户点击了三次炸弹,将显示GAME OVER信息,并重置窗口。
clock.tick()将保持循环以正确的速度运行。循环应该在每1/12秒后更新一次

推荐阅读:
入门: 最全的零基础学Python的问题 | 零基础学了8个月的Python | 实战项目 |学Python就是这条捷径
干货:爬取豆瓣短评,电影《后来的我们》 | 38年NBA最佳球员分析 | 从万众期待到口碑扑街!唐探3令人失望 | 笑看新倚天屠龙记 | 灯谜答题王 |用Python做个海量小姐姐素描图 |碟中谍这么火,我用机器学习做个迷你推荐系统电影
趣味:弹球游戏 | 九宫格 | 漂亮的花 | 两百行Python《天天酷跑》游戏!
AI: 会做诗的机器人 | 给图片上色 | 预测收入 | 碟中谍这么火,我用机器学习做个迷你推荐系统电影
小工具: Pdf转Word,轻松搞定表格和水印! | 一键把html网页保存为pdf!| 再见PDF提取收费! | 用90行代码打造最强PDF转换器,word、PPT、excel、markdown、html一键转换 | 制作一款钉钉低价机票提示器! |60行代码做了一个语音壁纸切换器天天看小姐姐!|
点阅读原文,看B站我的视频!