Python制作一款简易音乐播放器
Crossin的编程教室
共 6947字,需浏览 14分钟
· 2021-05-11
安装Python并添加到环境变量,pip安装相关模块:
pip install pyqt5
先睹为快
在cmd窗口运行"MusicPlayer.py"文件即可。
原理简介
一、设计界面
界面设计的比较简约,大概长这个样子:
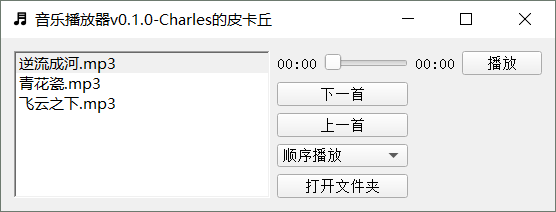
# 界面元素
# --播放时间
self.label1 = QLabel('00:00')
self.label1.setStyle(QStyleFactory.create('Fusion'))
self.label2 = QLabel('00:00')
self.label2.setStyle(QStyleFactory.create('Fusion'))
# --滑动条
self.slider = QSlider(Qt.Horizontal, self)
self.slider.sliderMoved[int].connect(lambda: self.player.setPosition(self.slider.value()))
self.slider.setStyle(QStyleFactory.create('Fusion'))
# --播放按钮
self.play_button = QPushButton('播放', self)
self.play_button.clicked.connect(self.playMusic)
self.play_button.setStyle(QStyleFactory.create('Fusion'))
# --上一首按钮
self.preview_button = QPushButton('上一首', self)
self.preview_button.clicked.connect(self.previewMusic)
self.preview_button.setStyle(QStyleFactory.create('Fusion'))
# --下一首按钮
self.next_button = QPushButton('下一首', self)
self.next_button.clicked.connect(self.nextMusic)
self.next_button.setStyle(QStyleFactory.create('Fusion'))
# --打开文件夹按钮
self.open_button = QPushButton('打开文件夹', self)
self.open_button.setStyle(QStyleFactory.create('Fusion'))
self.open_button.clicked.connect(self.openDir)
# --显示音乐列表
self.qlist = QListWidget()
self.qlist.itemDoubleClicked.connect(self.doubleClicked)
self.qlist.setStyle(QStyleFactory.create('windows'))
# --如果有初始化setting, 导入setting
self.loadSetting()
# --播放模式
self.cmb = QComboBox()
self.cmb.setStyle(QStyleFactory.create('Fusion'))
self.cmb.addItem('顺序播放')
self.cmb.addItem('单曲循环')
self.cmb.addItem('随机播放')
# --计时器
self.timer = QTimer(self)
self.timer.start(1000)
self.timer.timeout.connect(self.playByMode)
# 界面布局
self.grid = QGridLayout()
self.setLayout(self.grid)
self.grid.addWidget(self.qlist, 0, 0, 5, 10)
self.grid.addWidget(self.label1, 0, 11, 1, 1)
self.grid.addWidget(self.slider, 0, 12, 1, 1)
self.grid.addWidget(self.label2, 0, 13, 1, 1)
self.grid.addWidget(self.play_button, 0, 14, 1, 1)
self.grid.addWidget(self.next_button, 1, 11, 1, 2)
self.grid.addWidget(self.preview_button, 2, 11, 1, 2)
self.grid.addWidget(self.cmb, 3, 11, 1, 2)
self.grid.addWidget(self.open_button, 4, 11, 1, 2)
(1)存放音乐的文件夹选取
直接调pyqt5相应的函数就行:
'''打开文件夹'''
def openDir(self):
self.cur_path = QFileDialog.getExistingDirectory(self, "选取文件夹", self.cur_path)
if self.cur_path:
self.showMusicList()
self.cur_playing_song = ''
self.setCurPlaying()
self.label1.setText('00:00')
self.label2.setText('00:00')
self.slider.setSliderPosition(0)
self.is_pause = True
self.play_button.setText('播放')
打开文件夹后把所有的音乐文件显示在界面左侧,并保存一些必要的信息:
'''显示文件夹中所有音乐'''
def showMusicList(self):
self.qlist.clear()
self.updateSetting()
for song in os.listdir(self.cur_path):
if song.split('.')[-1] in self.song_formats:
self.songs_list.append([song, os.path.join(self.cur_path, song).replace('\\', '/')])
self.qlist.addItem(song)
self.qlist.setCurrentRow(0)
if self.songs_list:
self.cur_playing_song = self.songs_list[self.qlist.currentRow()][-1]
(2)音乐播放
音乐播放功能直接调用QMediaPlayer实现:
'''播放音乐'''
def playMusic(self):
if self.qlist.count() == 0:
self.Tips('当前路径内无可播放的音乐文件')
return
if not self.player.isAudioAvailable():
self.setCurPlaying()
if self.is_pause or self.is_switching:
self.player.play()
self.is_pause = False
self.play_button.setText('暂停')
elif (not self.is_pause) and (not self.is_switching):
self.player.pause()
self.is_pause = True
self.play_button.setText('播放')
点击上一首/下一首按钮切换:
'''上一首'''
def previewMusic(self):
self.slider.setValue(0)
if self.qlist.count() == 0:
self.Tips('当前路径内无可播放的音乐文件')
return
pre_row = self.qlist.currentRow()-1 if self.qlist.currentRow() != 0 else self.qlist.count() - 1
self.qlist.setCurrentRow(pre_row)
self.is_switching = True
self.setCurPlaying()
self.playMusic()
self.is_switching = False
'''下一首'''
def nextMusic(self):
self.slider.setValue(0)
if self.qlist.count() == 0:
self.Tips('当前路径内无可播放的音乐文件')
return
next_row = self.qlist.currentRow()+1 if self.qlist.currentRow() != self.qlist.count()-1 else 0
self.qlist.setCurrentRow(next_row)
self.is_switching = True
self.setCurPlaying()
self.playMusic()
self.is_switching = False
双击某首歌切换:
'''双击播放音乐'''
def doubleClicked(self):
self.slider.setValue(0)
self.is_switching = True
self.setCurPlaying()
self.playMusic()
self.is_switching = False
根据播放模式切换:
'''根据播放模式播放音乐'''
def playByMode(self):
if (not self.is_pause) and (not self.is_switching):
self.slider.setMinimum(0)
self.slider.setMaximum(self.player.duration())
self.slider.setValue(self.slider.value() + 1000)
self.label1.setText(time.strftime('%M:%S', time.localtime(self.player.position()/1000)))
self.label2.setText(time.strftime('%M:%S', time.localtime(self.player.duration()/1000)))
# 顺序播放
if (self.cmb.currentIndex() == 0) and (not self.is_pause) and (not self.is_switching):
if self.qlist.count() == 0:
return
if self.player.position() == self.player.duration():
self.nextMusic()
# 单曲循环
elif (self.cmb.currentIndex() == 1) and (not self.is_pause) and (not self.is_switching):
if self.qlist.count() == 0:
return
if self.player.position() == self.player.duration():
self.is_switching = True
self.setCurPlaying()
self.slider.setValue(0)
self.playMusic()
self.is_switching = False
# 随机播放
elif (self.cmb.currentIndex() == 2) and (not self.is_pause) and (not self.is_switching):
if self.qlist.count() == 0:
return
if self.player.position() == self.player.duration():
self.is_switching = True
self.qlist.setCurrentRow(random.randint(0, self.qlist.count()-1))
self.setCurPlaying()
self.slider.setValue(0)
self.playMusic()
self.is_switching = False
代码文件
公众号内回复关键字:音乐播放器
作者:Charles未晞
_往期文章推荐_
评论
Python列表知识应知应会
点击上方“Go语言进阶学习”,进行关注回复“Go语言”即可获赠从入门到进阶共10本电子书今日鸡汤只在此山中,云深不知处。一、前言 在Python程序开发中,列表(List)经常会使用。假设一个班里有50个学生现需要统计每一个学生的总成绩情况,如果不使用列
Go语言进阶学习
0
Python 字符串应该用双引号还是单引号?
转载来源:洪尔摩斯PyCharm升级至 2023.2版本后,经常弹出来一个提示问我要不要试一下Black formatter。试了一下,这个Black formatter 很有个性,特别喜欢换行。我的一个文件用PyCharm自带的代码整理器整理完之后是500行左右,然后再用Black整理就变成600
菜鸟学Python
0
delorean,一个超级实用的 Python 库!
作者通常周更,为了不错过更新,请点击上方“Python碎片”,“星标”公众号大家好,今天为大家分享一个超级实用的 Python 库 - delorean。Github地址:https://github.com/myusuf3/delorean/时间在计算机科学和软件开发中是一个至关重要的概念。Pyt
Python 碎片
0
五一Python抢票神器来了
还在为五一回家抢不到火车票发愁吗?今天介绍一个Python抢票神器,希望对你有帮助。Py12306是一个流行的开源项目,旨在帮助用户更便捷地查询和预订中国铁路12306网站上的火车票。以下是使用Py12306的基本步骤和一些注意事项:安装与环境准备安装Python: 确保你的系统中安装了Python
Python小二
1
PyPy为什么能让Python比C还快?一文了解内在机制
我的小册:(小白零基础用Python量化股票分析小册) ,原价299,限时特价2杯咖啡,满100人涨10元。来源:机器之心「如果想让代码运行得更快,您应该使用 PyPy。」—— Python 之父 Guido van Rossum对于研究人员来说,迅速把想法代码化并查看其是否行得通至关重要。Pyth
菜鸟学Python
0
CleverCSV,一个神奇的 python 库!
我的小册:(小白零基础用Python量化股票分析小册) ,原价299,限时特价2杯咖啡,满100人涨10元。来源丨网络介绍CleverCSV 是一个基于 Python 的库,旨在提供比标准库 csv 更智能和灵活的方法来处理 CSV 文件。该库使用机器学习算法来探测 CSV 文件的正确拨号结构,从而
菜鸟学Python
0
python读取多个excel表多个sheet后映射匹配再分组计算、纵向拼接
大家好,我是飞奔的蜗牛ing。一、前言前几天在一个客户给到一单数据处理的 问题,需求是这样的:1.表“aa2020”中2020年数据需要按季度分成四个表。(1-3月、4-6月、7-9月、10-12月)2.表“2020年一季度”代表2020年一季度客户所对应的管理档位,需要把表中的档位导入附表“aa2
IT共享之家
0
Java项目实战——打造一款股票区间交易盯盘系统
点击上方“Java进阶学习交流”,进行关注后台回复“Java”即可获赠Java学习资料今日鸡汤身无彩凤双飞翼,心有灵犀一点通。一、简介大家好,我是Snowball。今天给大家分享的内容是基于Java编程,实现股票交易相关功能开发,如果读者对股票或金融衍生物交易不太了解,又比较感兴趣的话可自行查询相关
Java进阶学习交流
0